Stop spam in your forms in 2021
Adding Google reCAPTCHA to your forms prevents spam or bots from creating chaos in your mailbox. This will give you added security to your website without missing contact from your users.
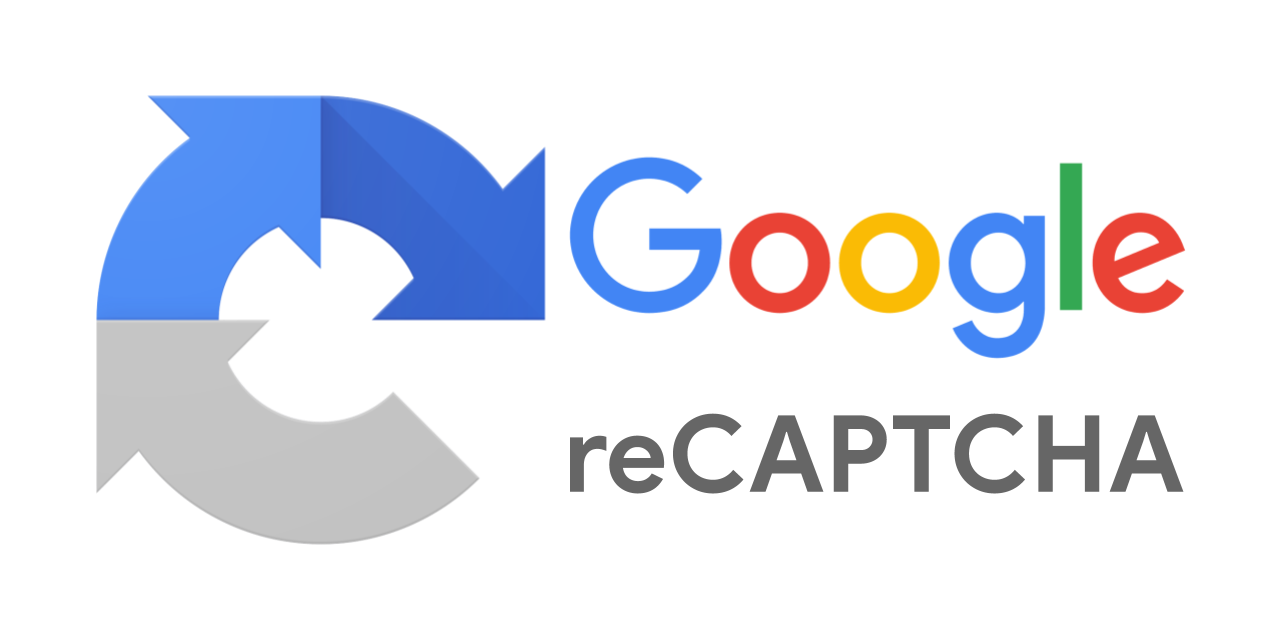
- Register your site with Google reCAPTCHA admin panel for v3 (getting site key and site secret)
- Create a basic contact form, with reCAPTCHA javascript library and site key (client side)
- Upon submission, send the response with the secret key to the server side for validation – if verified, send the email
Register with Google reCAPTCHA admin panel
Click the “Get Started” link and register for version 3. *Note: we will not be using the enterprise edition.- Register you site at Google reCAPTCHA Notice that you will need to register your localhost pr 127.0.0.1 (IPv4) :: 1 (IPv6) if you want to test the forms on a local environment. Make sure to register the domain of your site.


The contact form
Create a basic html file or php file to hold the form. The contact form will have a few functions:- Notify user if the POST request has been successful or not
- Contain the input fields to collect from the user
- Include the Public site key in the form
< !-- (A) GOOGLE RECAPTCHA API -- >
<script src='https://www.google.com/recaptcha/api.js'>
</script>
< !-- (B) PROCESS AND NOTIFICATIONS ON CLIENT -- >
<?php
if (isset($_POST['name'])) {
require "process-form.php";
echo $error=="" ? "<div id='notify'>OK </div>"
: "<div id='notify'>$error </div>";
}
?>
<!-- (C) CONTACT FORM -->
<form id="contactForm" method="post">
<h1gt; Contact Us</h1>
<label>Your Name<label>
<input type="text" name="name" required />
<label>Email Address
<input type="email" name="email" required />
<label>Message
<textarea name="message" required></textarea>
<!-- (D) CAPTCHA - CHANGE SITE KEY! -- >
<div class="g-recaptcha" data-sitekey="SITE KEY"></div>
<input type="submit" value="Submit" />
</form>
Backend processing
Here we will do a few things: provide a process status, call on to verify that google api has processed the reCAPTCHA, and then send the email. We will utilizejson_decode()
with file_get_contents()
. json_decode()
will return the encoded JSON value in a php array.
<?php
// (A) PROCESS STATUS
$error = "";
// (B) VERIFY CAPTCHA
$secret = 'SECRET KEY'; // CHANGE THIS TO YOUR OWN!
$url = "https://www.google.com/recaptcha/api/siteverify?.
secret=$secret&response=".$_POST['g-recaptcha-response'];
$verify = json_decode(file_get_contents($url));
if (!$verify->success) { $error = "Invalid captcha"; }
// (C) SEND MAIL
if ($error=="") {
$mailTo = "john@doe.com"; // ! CHANGE THIS TO YOUR OWN !
$mailSubject = "Contact Form";
$mailBody = "";
foreach ($_POST as $k=>$v) {
if ($k!="g-recaptcha-response")
{ $mailBody .= "$k: $v\r\n"; }
}
if (!@mail($mailTo, $mailSubject, $mailBody))
{ $error = "Failed to send mail"; }
}
?>
Why is there no image for reCAPTCHA v3?
Unlike previous reCAPTCHA versions, this one does not require for you to identify any images. This version calculates a score from 0.0 to 0.9, with 0.9 score being most likely human, and anything closer to 0 is most likely a bot.
You can see your score distribution in the Goolge reCPATCHA admin page.

References: Code Prettify